Here's a result of what it can produce:
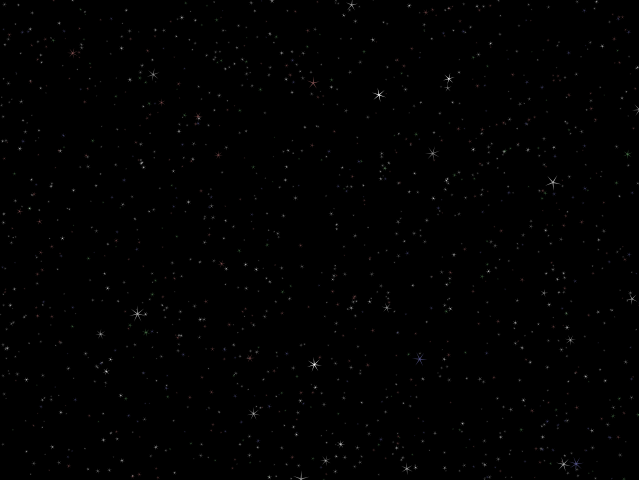
The jsFiddle is here: http://jsfiddle.net/TBMTT/
I'm using EaselJS as the HTML5 drawing library, although for the star field, the drawing can be achieved using the standard HTML5 canvas methods.
Below is the code used to generate the star field. It will generate 1500 stars with various sizes and colours.Most of the stars will be 'small', however there is a chance to get a 'big' star. This chance is quite low to make the field look more realistic. The colours are also chosen at random for all star sizes.
backgroundStage = new createjs.Stage("backgroundCanvas");
var stars = 1500;
var starField = new createjs.Shape();
var canvasWidth = 640;
var canvasHeight = 480;
var starSmallRadiusMin = 1;
var starSmallRadiusVarience = 2;
var starBigRadiusMin = 3;
var starBigRadiusVarience = 6;
var starBigChance = 0.025;
for (var i = 0; i < stars; i++) {
var mX = Math.floor(Math.random() * canvasWidth);
var mY = Math.floor(Math.random() * canvasHeight);
var bigStar = Math.random();
var radius;
if (bigStar < starBigChance) {
radius = starBigRadiusMin + (Math.random() * starBigRadiusVarience)
}
else {
radius = starSmallRadiusMin + (Math.random() * starSmallRadiusVarience);
}
var colour, colourType = Math.round(Math.random() * 5);
switch (colourType) {
case 0: colour = "#558"; break;
case 1: colour = "#fff"; break;
case 2: colour = "#aaa"; break;
case 3: colour = "#888"; break;
case 4: colour = "#855"; break;
case 5: colour = "#585"; break;
}
starField.graphics.beginFill(colour)
.drawPolyStar(
Math.random() * canvasWidth,
Math.random() * canvasHeight,
radius,
5 + Math.round(Math.random() * 2), // number of sides
0.9, // pointyness
Math.random() * 360 // rotation of the star
);
}
backgroundStage.addChild(starField);
backgroundStage.update();
-i