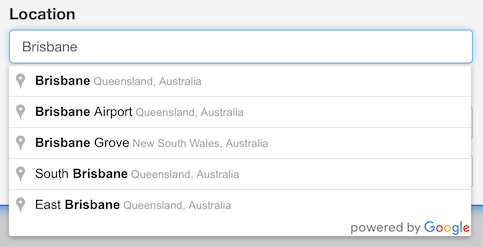
The issue I was trying so solve was that I needed geographic coordinates for a Place. The Places API of course lets you look this up, but that's a separate query and hence another round-trip to Google's servers. It turns out that when you get a Place via Autocomplete, it already has these coordinates. So instead of looking them up later all I had to do was store them somewhere.
The trick to getting hold of this data was adding an event listener for the 'place_changed' event and then storing it as user data into the input element that has Autocomplete enabled.
This is what the code looks like (see my earlier article I linked above for details on why there is an .each() call)...
JavaScript
$(document).ready(function() {
if (typeof(google) != 'undefined') {
var options = {
types: ['(cities)']
};
$('input.loc_autocomplete').each(function(idx, el) {
var autoComplete = new google.maps.places.Autocomplete(el, options);
/* add a reference back to the input element */
autoComplete.inputEl = el;
/* add event listener */
google.maps.event.addListener(autoComplete, 'place_changed', function() {
var loc = this.getPlace().geometry.location;
/* store the location as user data */
$(this.inputEl).data({lat: loc.lat(), lng: loc.lng()});
});
});
}
});
The code is more or less the same as what I've shared in my previous article, with a few additions. On line 11 I create a reference back to the input element for which I am adding Autocomplete for. This is required to store the user data inside the listener.
Lines 14-18 have the event listener. This code gets the coordinates of the Place and adds them to the original input element's data attributes.
To access these coordinates later, all I have to do is look up its data()...
JavaScript
var coords = $(myInput).data();
console.log('Latitude: ' + coords.lat);
console.log('Longitude: ' + coords.lng);
-i